You can execute Sikuli Slides using JRuby. The helloworld program only takes two lines:
# helloworld.rb
require 'sikuli-slides'
Sikuli::Slides.execute "helloworld.pptx"
Installation
You must first install JRuby and RubyGems on your system. Then, you can do the following to install Sikuli Slides and run the helloworld program.
Install the Sikuli Slides Gem. It will download and install everything you need.
$ jruby -S gem install sikuli-slides
Tell JRuby to run in the 1.9 mode
$ export JRUBY_OPTS=--1.9
Run helloworld.rb
$ jruby helloworld.rb
Parameters
Sikuli::Slides.execute
is the main entry point of Sikuli Slides’s JRuby API. You can provide extra hash arguments to control various aspects of the execution.
# Execute with the parameter <user>'s value set to "Sikuli"
Sikuli::Slides.execute "hellouser.pptx", :params => {:user => "Sikuli"}
# Execute on another screen (id = 1)
Sikuli::Slides.execute "10steps.pptx", :screen => 1
# Execute starting from slide number 5 (one-based)
Sikuli::Slides.execute "10steps.pptx", :start => 5
# Execute starting from the slide that has the bookmark 'foo'
Sikuli::Slides.execute "10steps.pptx", :start => "foo"
# Execute with the minimum similarity score set to 0.9
Sikuli::Slides.execute "10steps.pptx", :minScore => 0.9
# Execute with the time to wait for the next target to 3000ms
Sikuli::Slides.execute "10steps.pptx", :waitTime => 3000
# Execute with the log level set to OFF
# other options are: (ALL, TRACE, DEBUG, INFO, WARN, ERROR, OFF)
Sikuli::Slides.execute "10steps.pptx", :logLevel => 'OFF'
Example: Currency Conversion
Code
require 'sikuli-slides'
# location to the online currency converter
Sikuli::API.browse "http://www.xe.com/currencyconverter/#converter"
# location to download slides
slidesUrl = "http://slides.sikuli.org/examples/player/xe/convert.pptx"
# execute the slides 10 times
10.times do
# each time, a random number between 1 and 1000 is generated
x = (1..1000).to_a.sample
# this number is given as a parameter to the slides
Sikuli::Slides.execute slidesUrl, :params => {:amount => x}
end
Slides
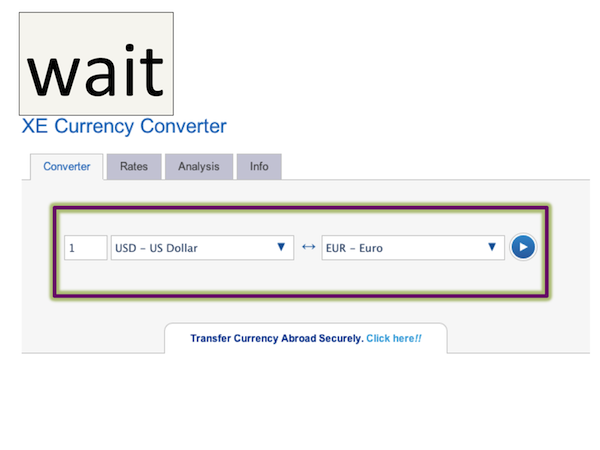
Slide 1
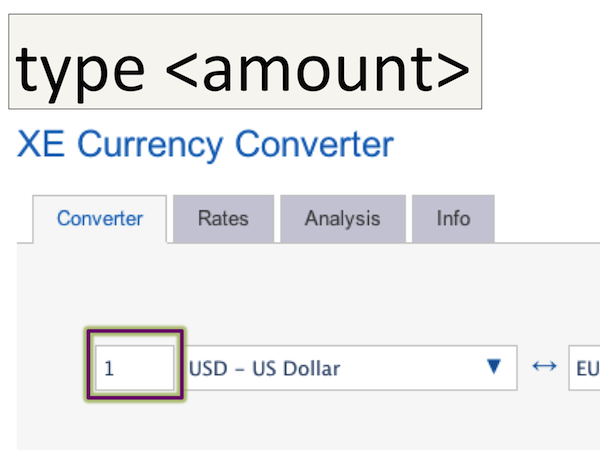
Slide 2
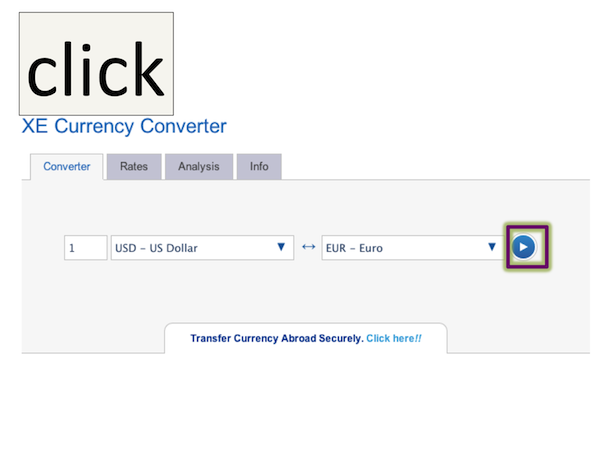
Slide 3
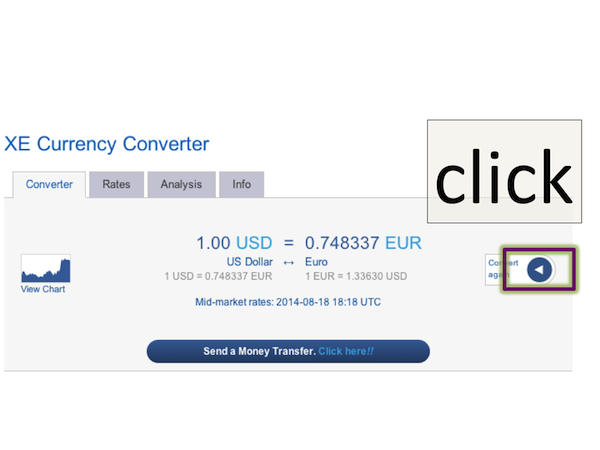
Slide 4
Example: Hello Adam, Ben, Cindy
Code
hellouser.pptx
["Adam","Ben","Cindy"].each do |name|
Sikuli::Slides.execute "hellouser.pptx", :params => {:user => name}
end
Slides
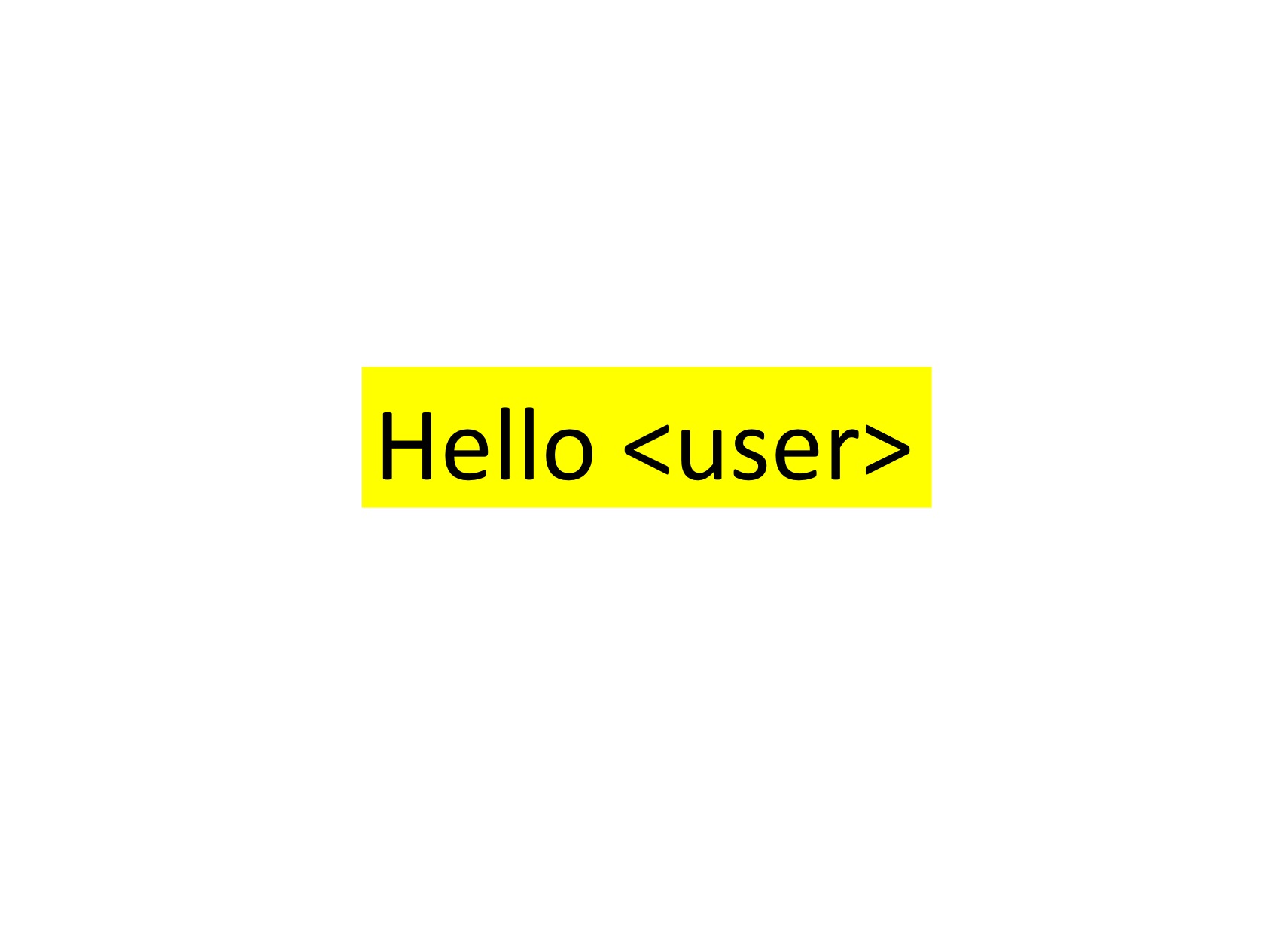